Agora Voice & Video calls with cloud functions in flutter
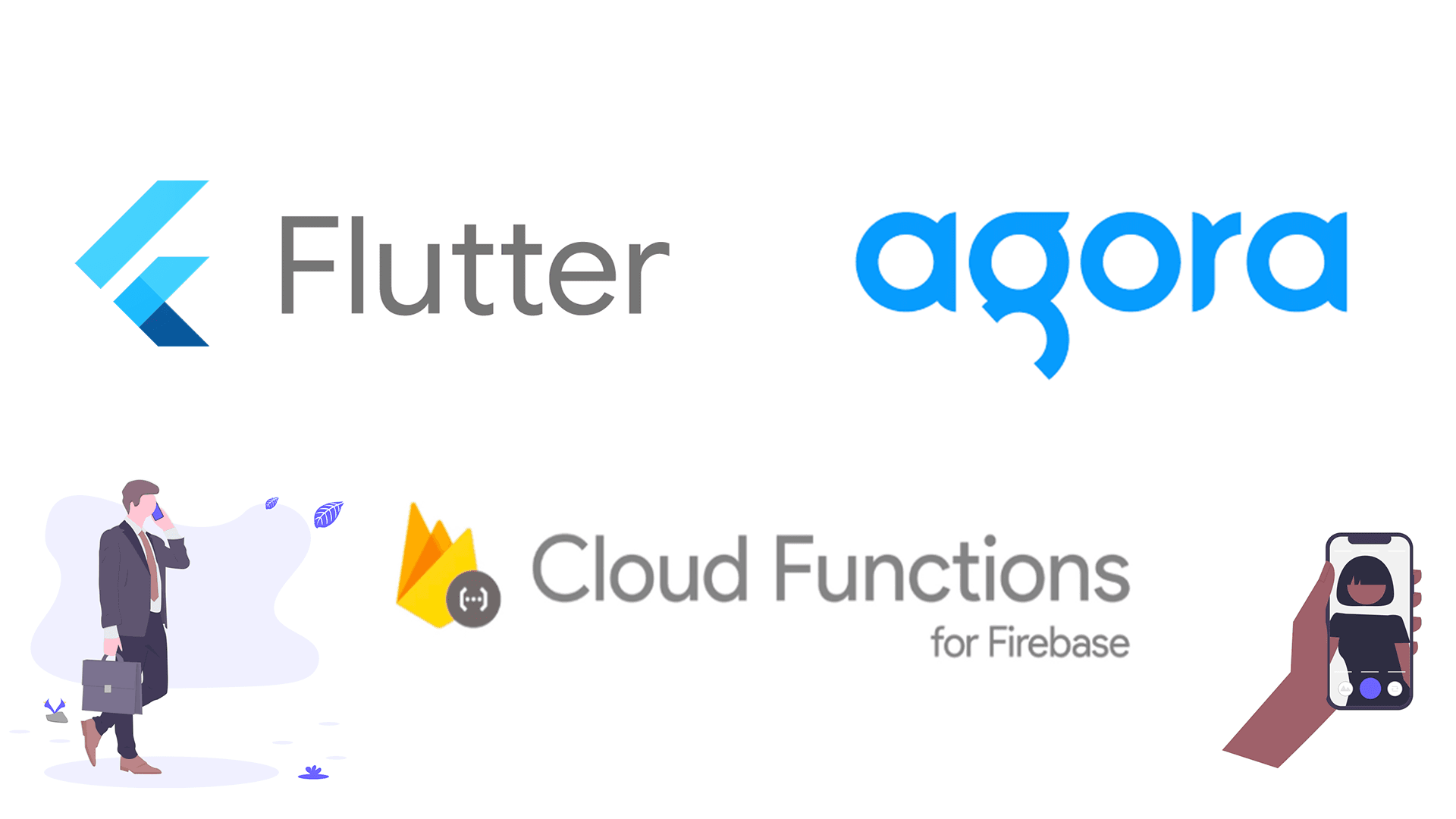
In this article I am going to teach you how to implement voice & video calls in flutter application using agora-rtc-engine. In agora sdk we have to create a token for a particular call and we are going to do that using firebase cloud functions.
Step1- Quick Set-Up- Integrating Flutter App With Firebase ( You can do it by yourself)
Step2 — Enable cloud functions in firebase project
To use firebase cloud functions in your project first you have to upgrade your project to a blaze project.
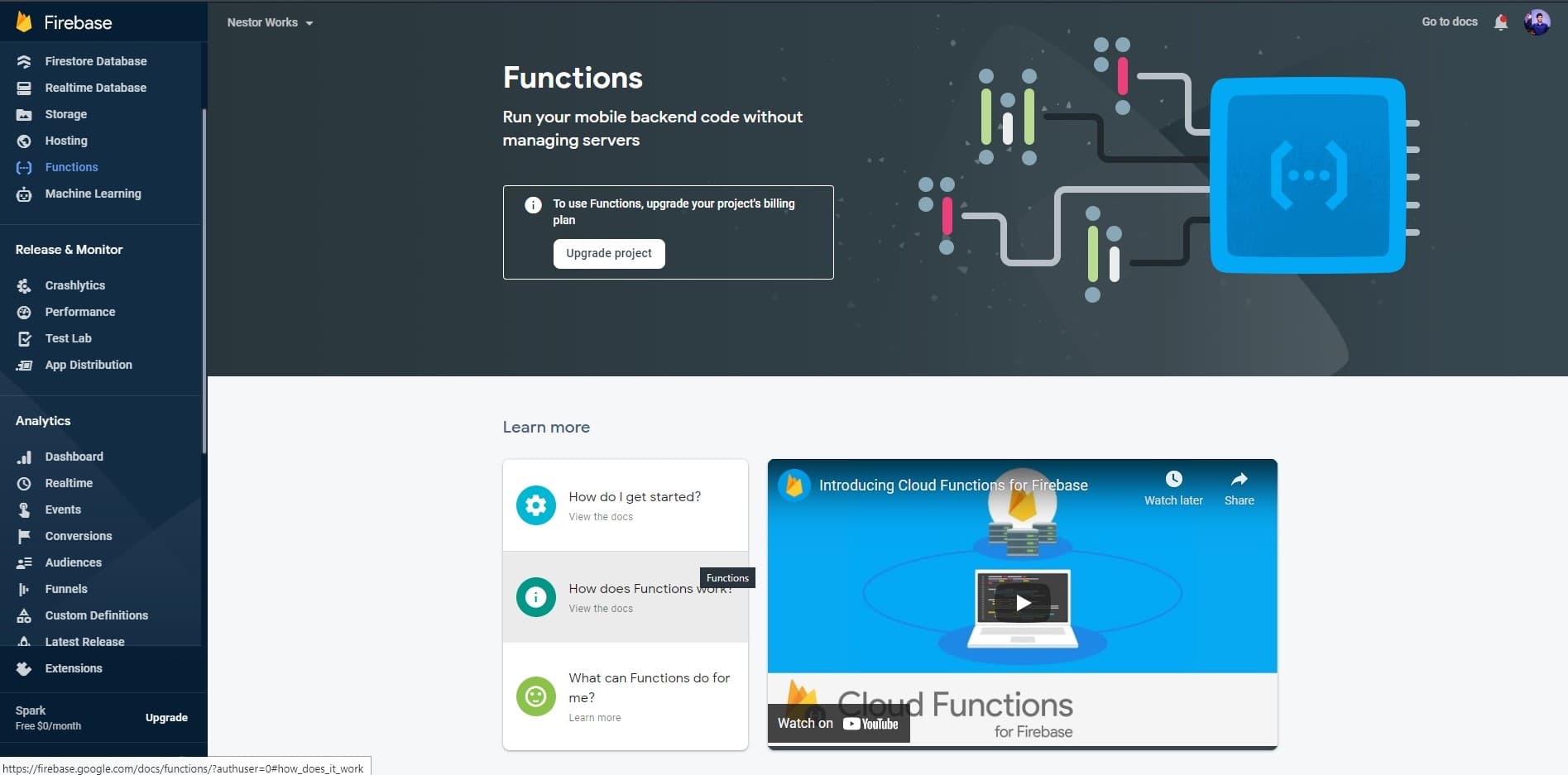
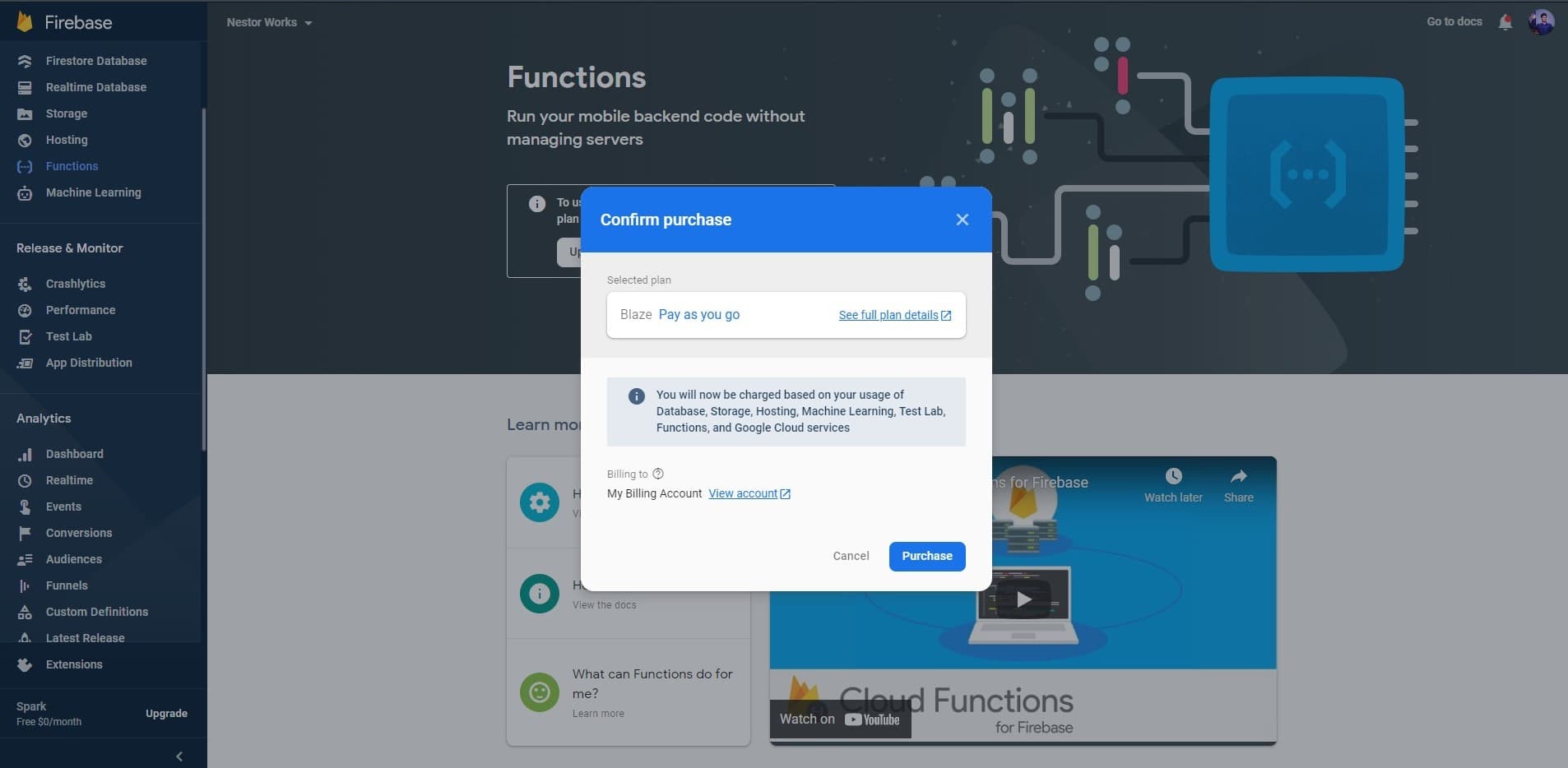
After upgrading your project to the blaze then go to project settings. Click the service account tab and then generate a new private key. After that download the service account json file.
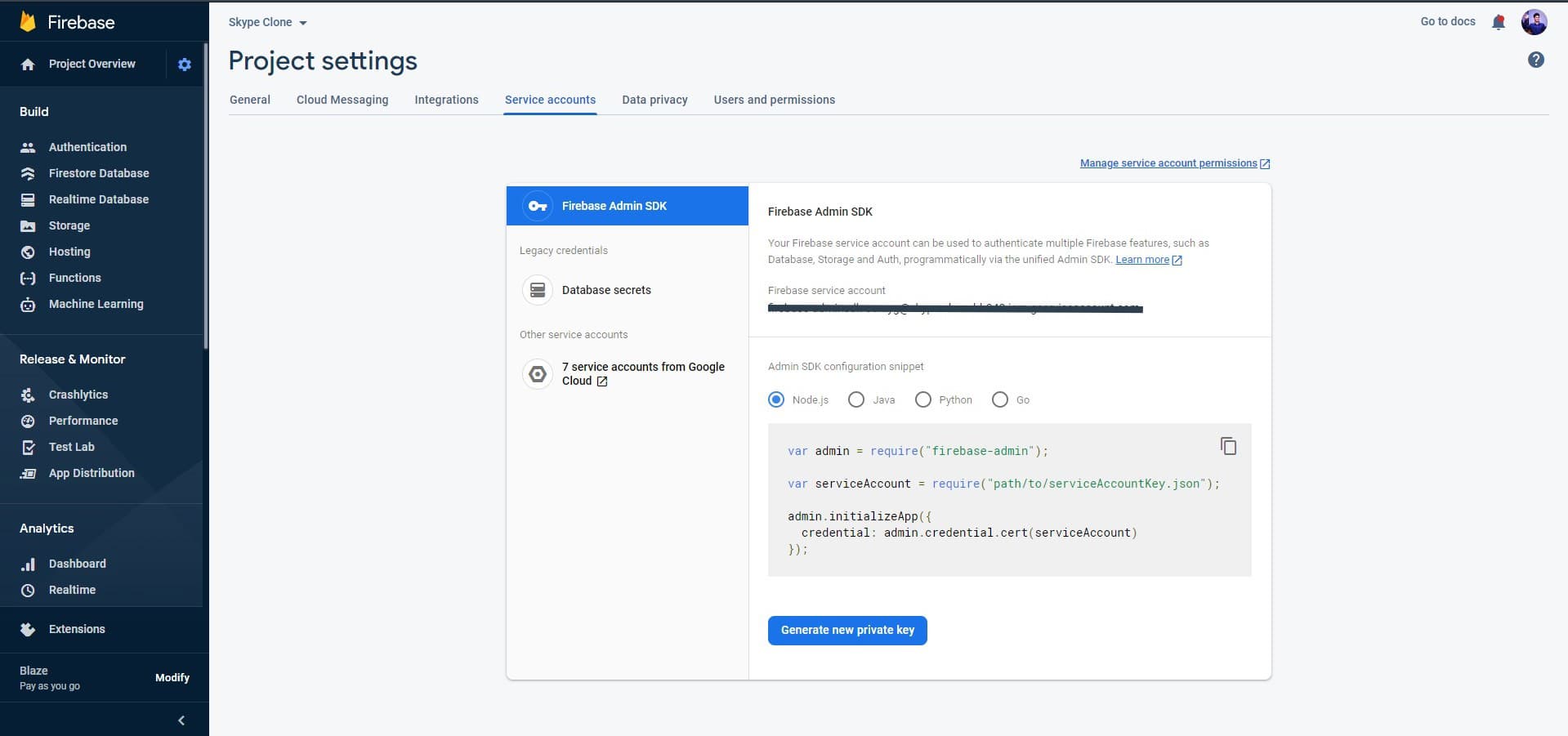
Step3 - Install dependencies in flutter project
First of all create a new folder in your flutter project root called “Firebase-Functions” and inside that folder open the command prompt and type “firebase init” and complete the process shown in below.
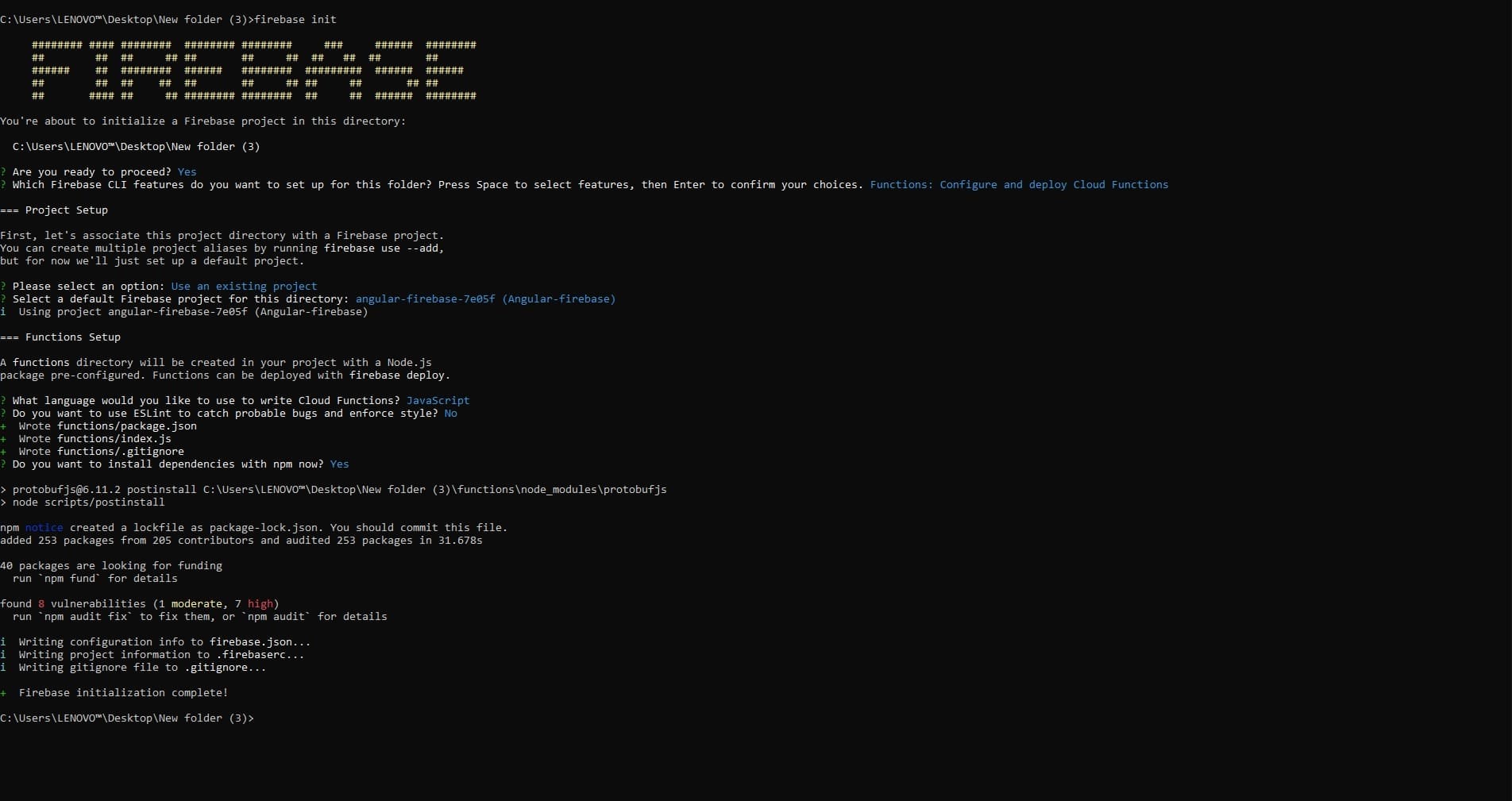
After complete that part there should be some files in your “ firebase-functions” folder as below.
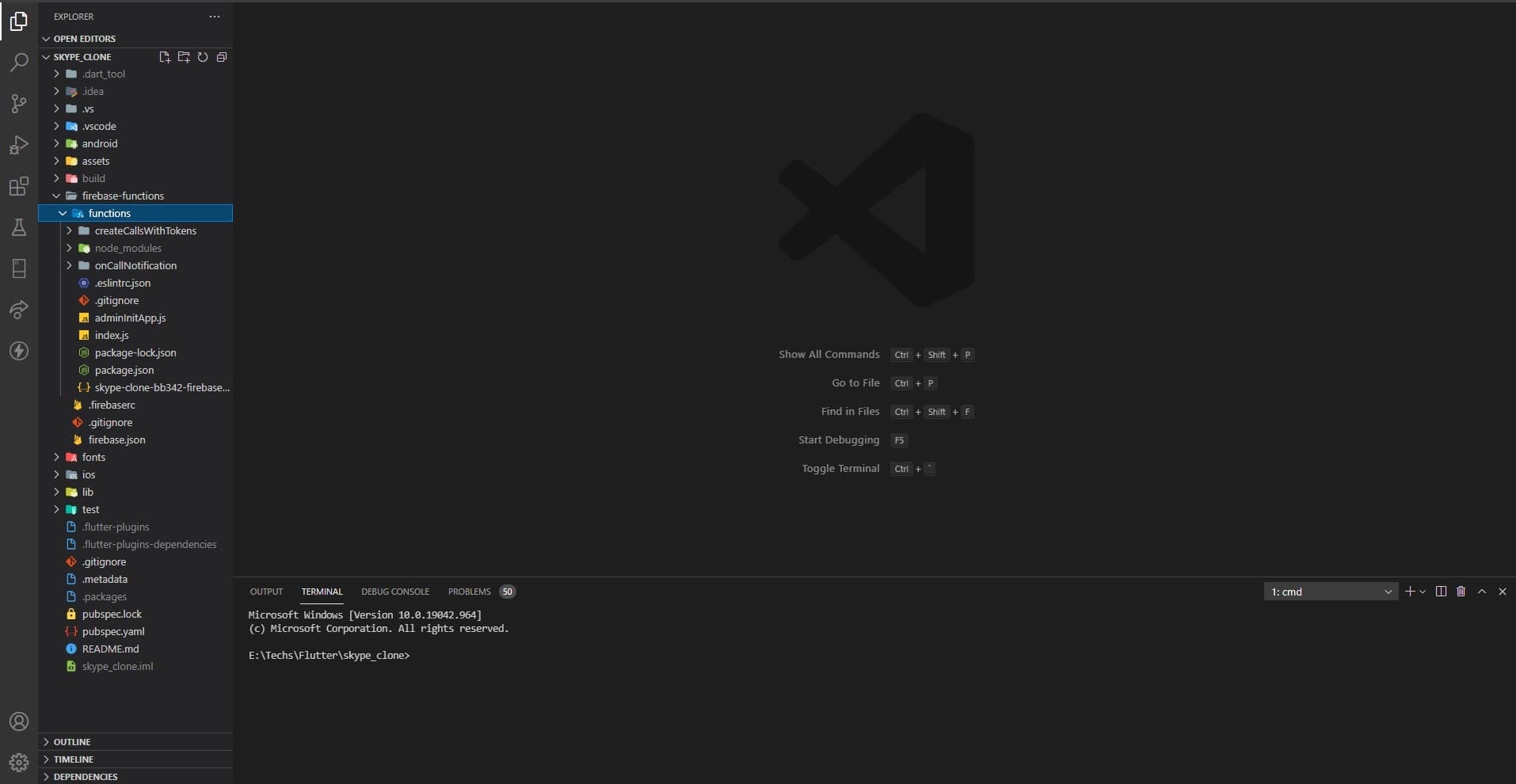
Then go to the functions folder and install “firebase-admin”, “firebase-functions” and “agora-access-token” dependencies to your project using,
- npm install firebase-admin
- npm install firebase functions
- npm install agora-access-token
Step4- Create a project in agora.io
Go to agora.io and login to the dashboard. Then go to the overview and create a new project.
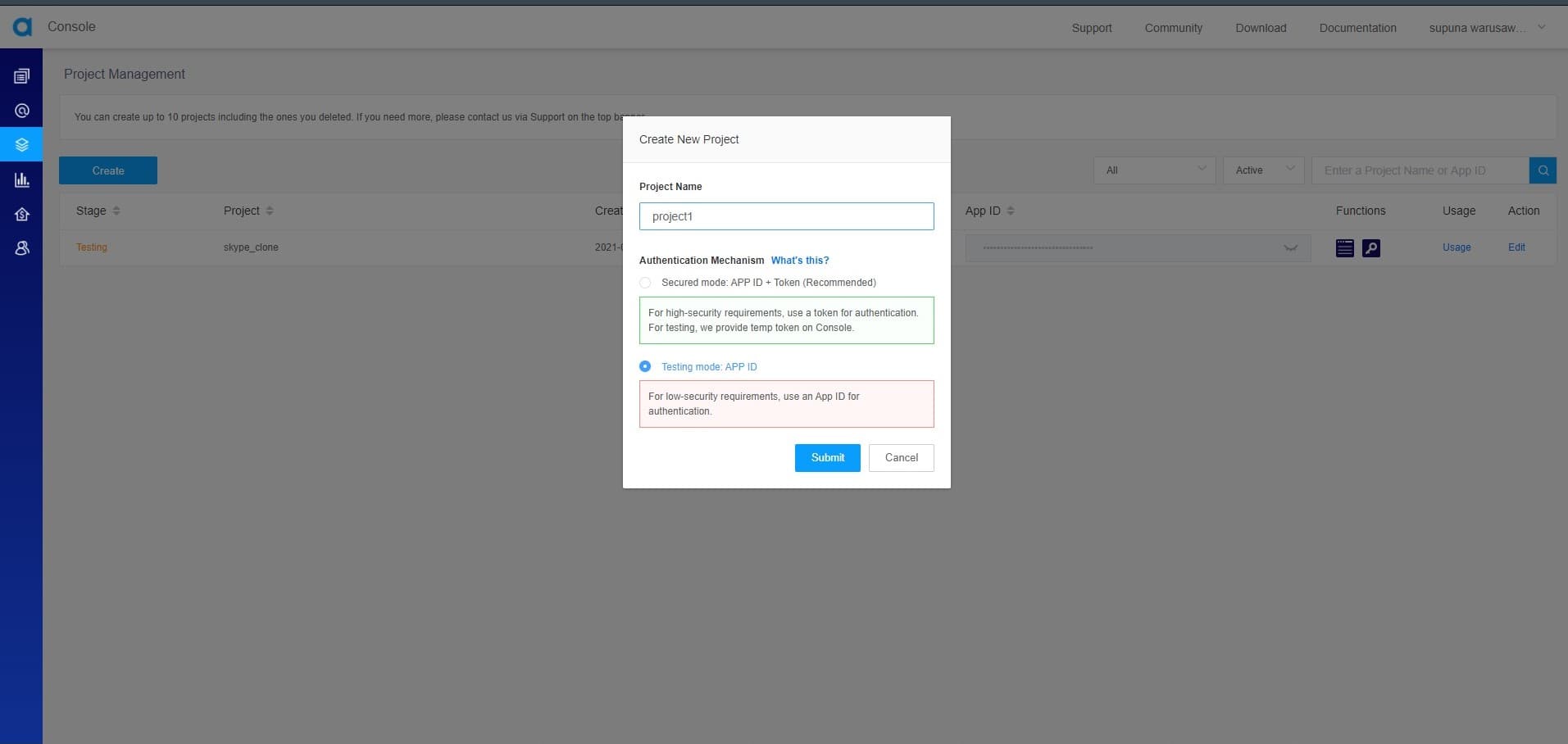
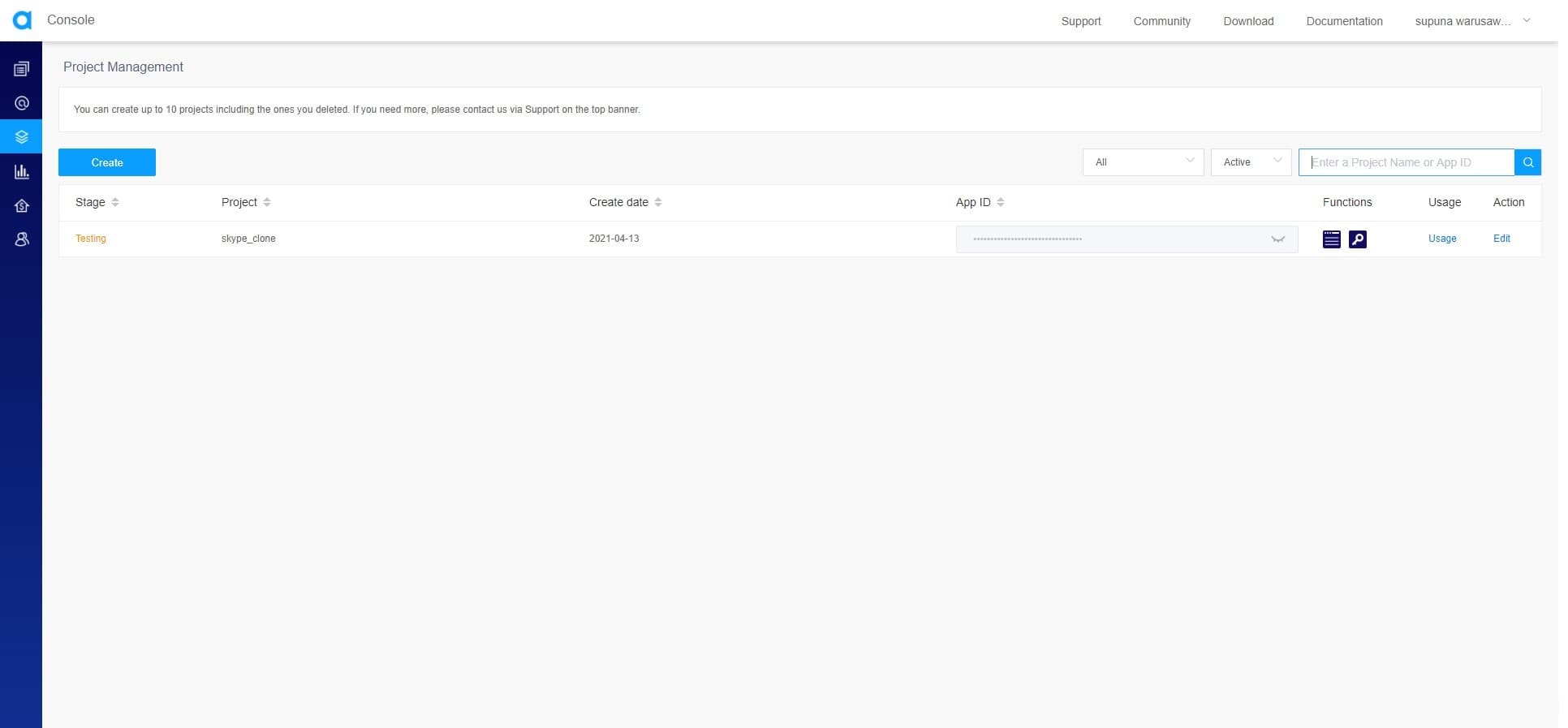
Now edit the project settings and enable secondary certificate.
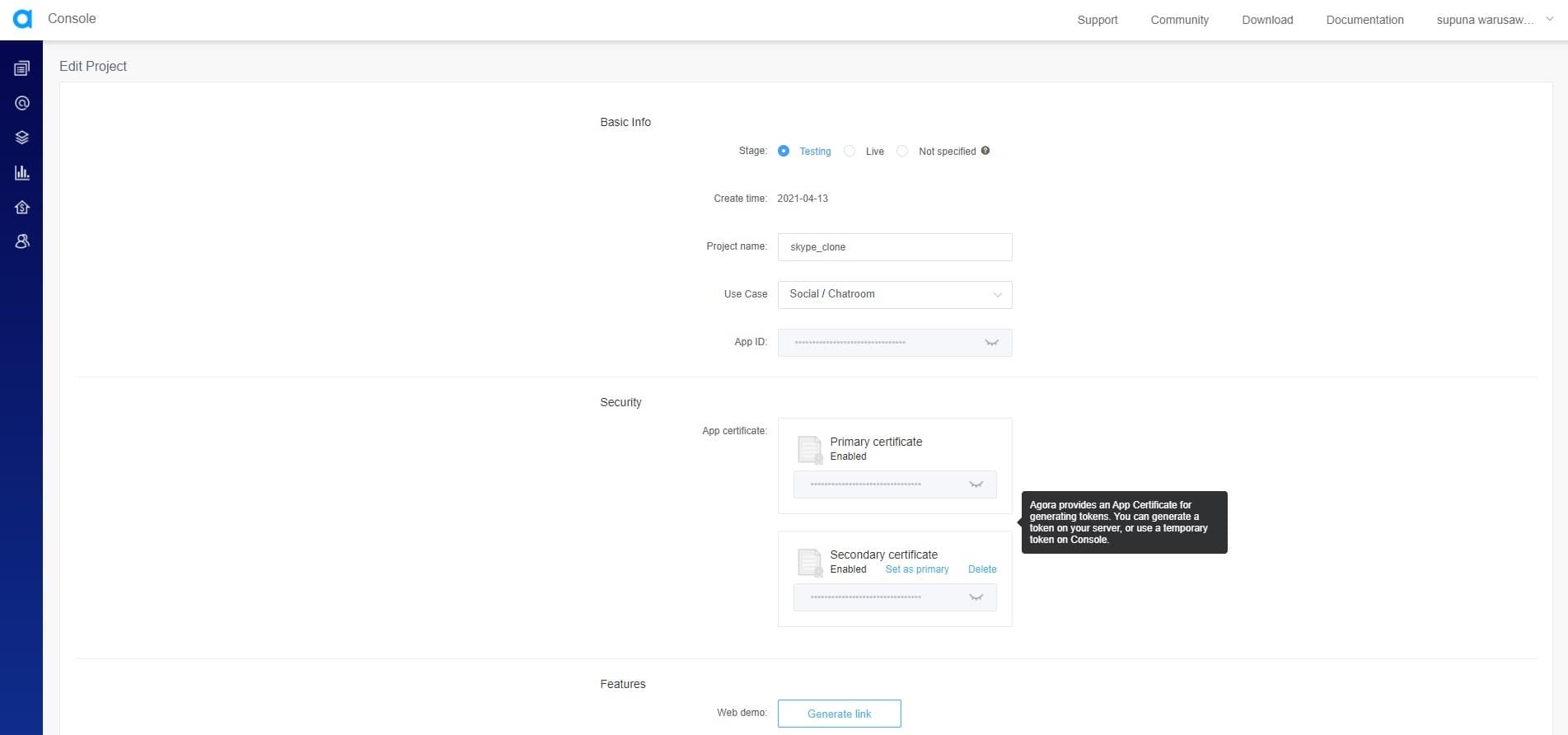
Then you have to copy appId, appCertificate to the createCallsWithToken.js file.
Step5- Create our first cloud function
Now you have to copy the downloaded service account json file into this functions folder. After that create a index.js file and adminInitApp.js file inside that folder. Then create a new folder called “createCallsWithTokens” and add a new createCallsWithTokens.js file inside that.
adminInitApp.js
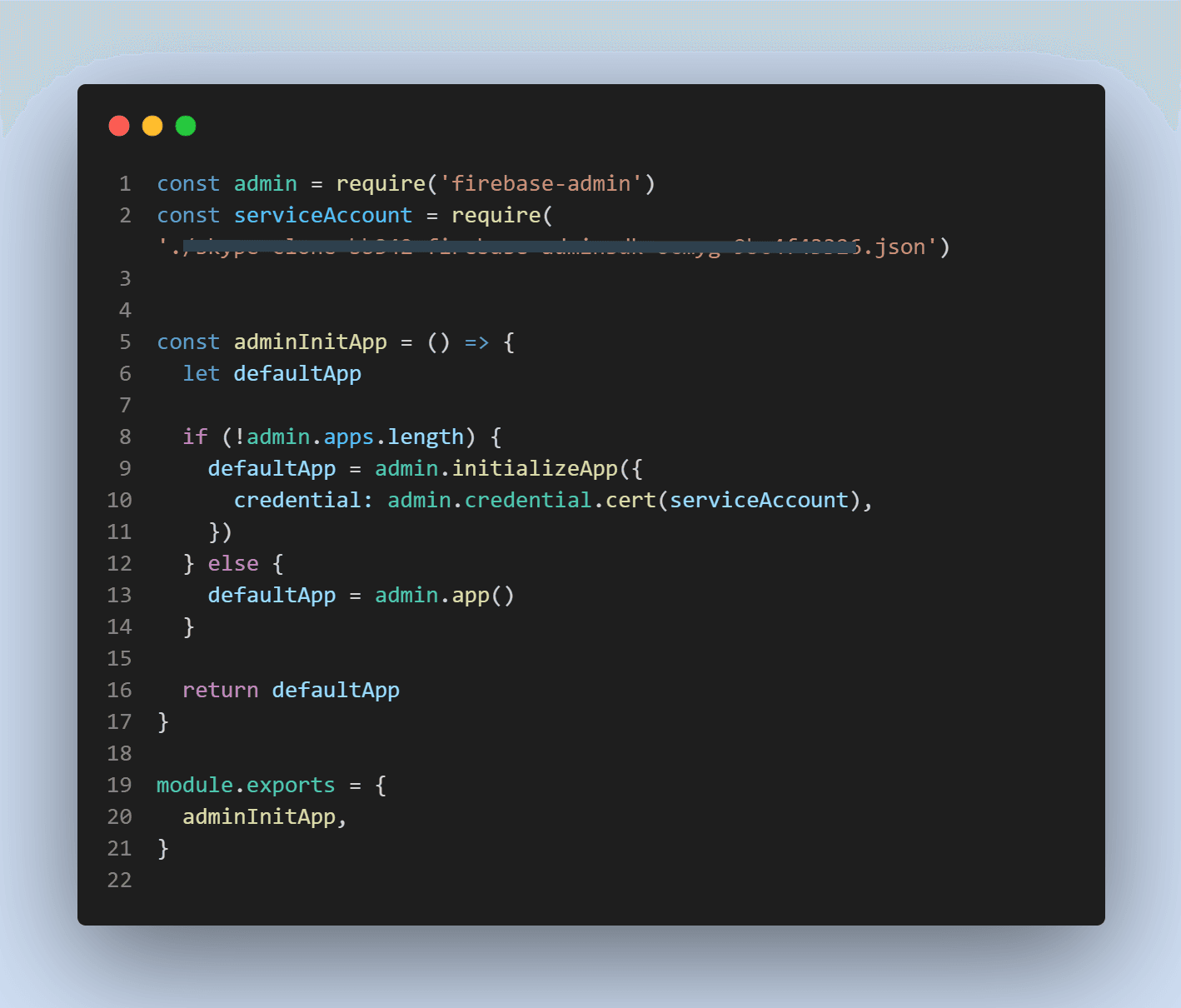
index.js
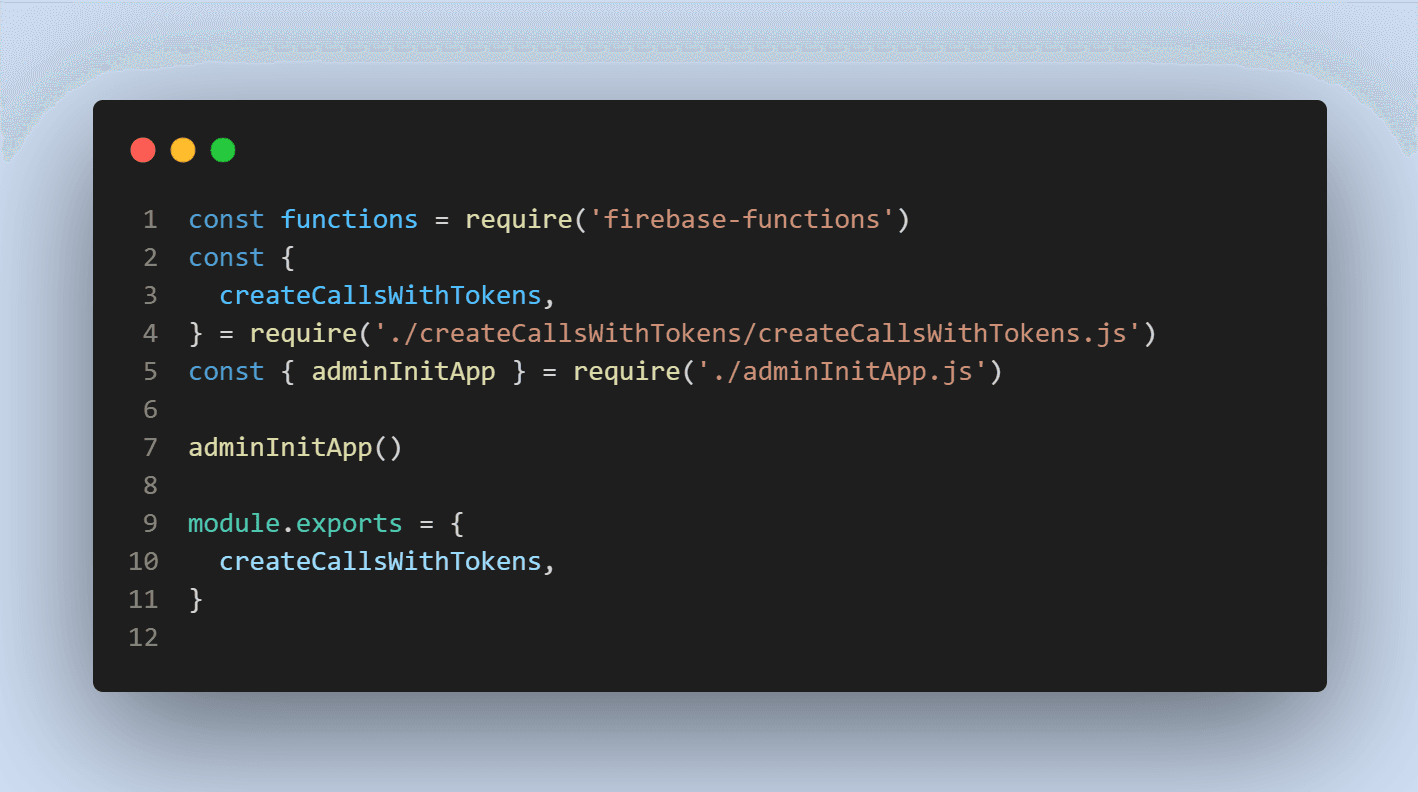
createCallsWithToken.js
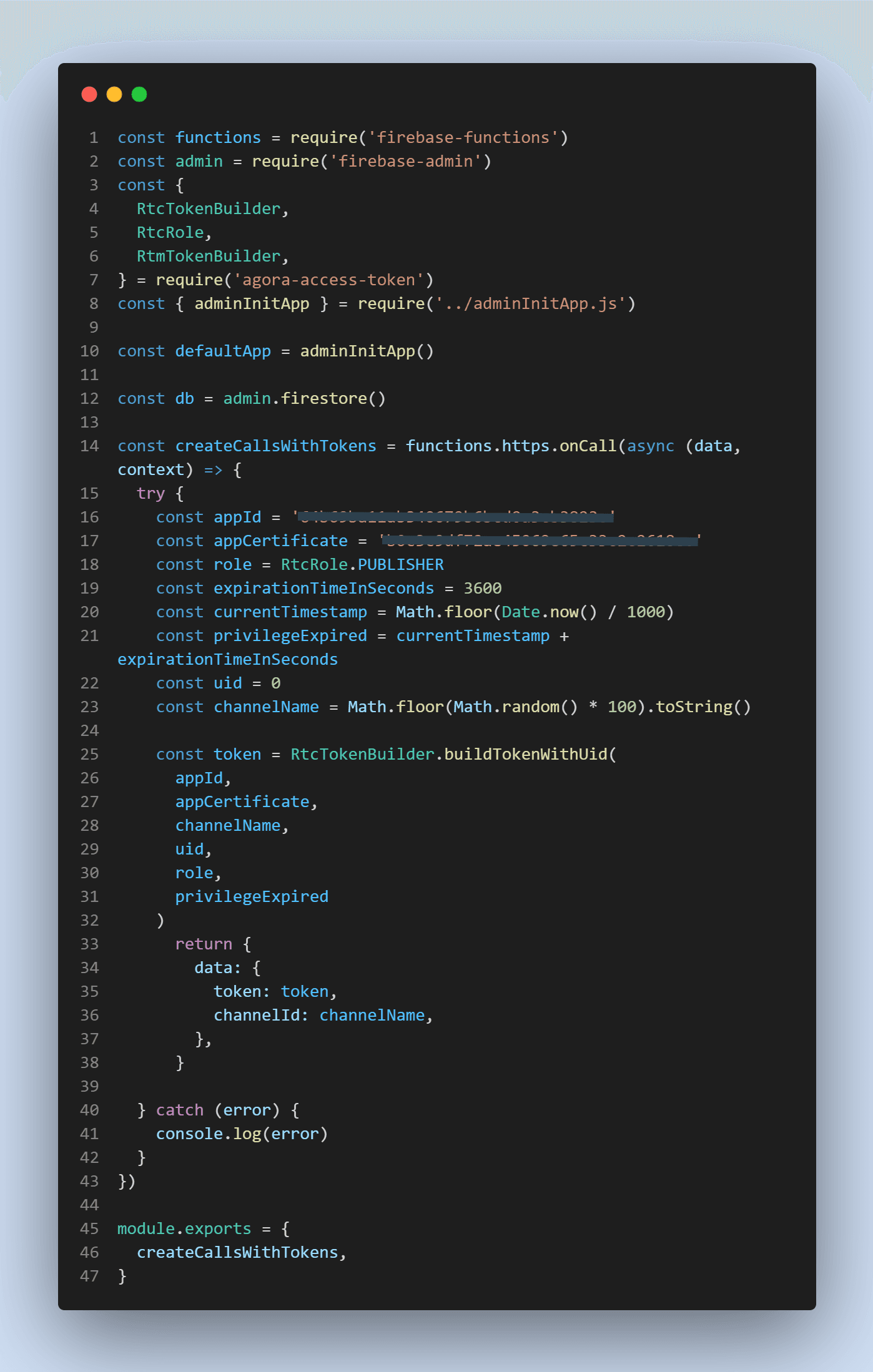
Finally go to the firebase-functions folder and open the command prompt and deploy functions using “firebase deploy — only functions”.
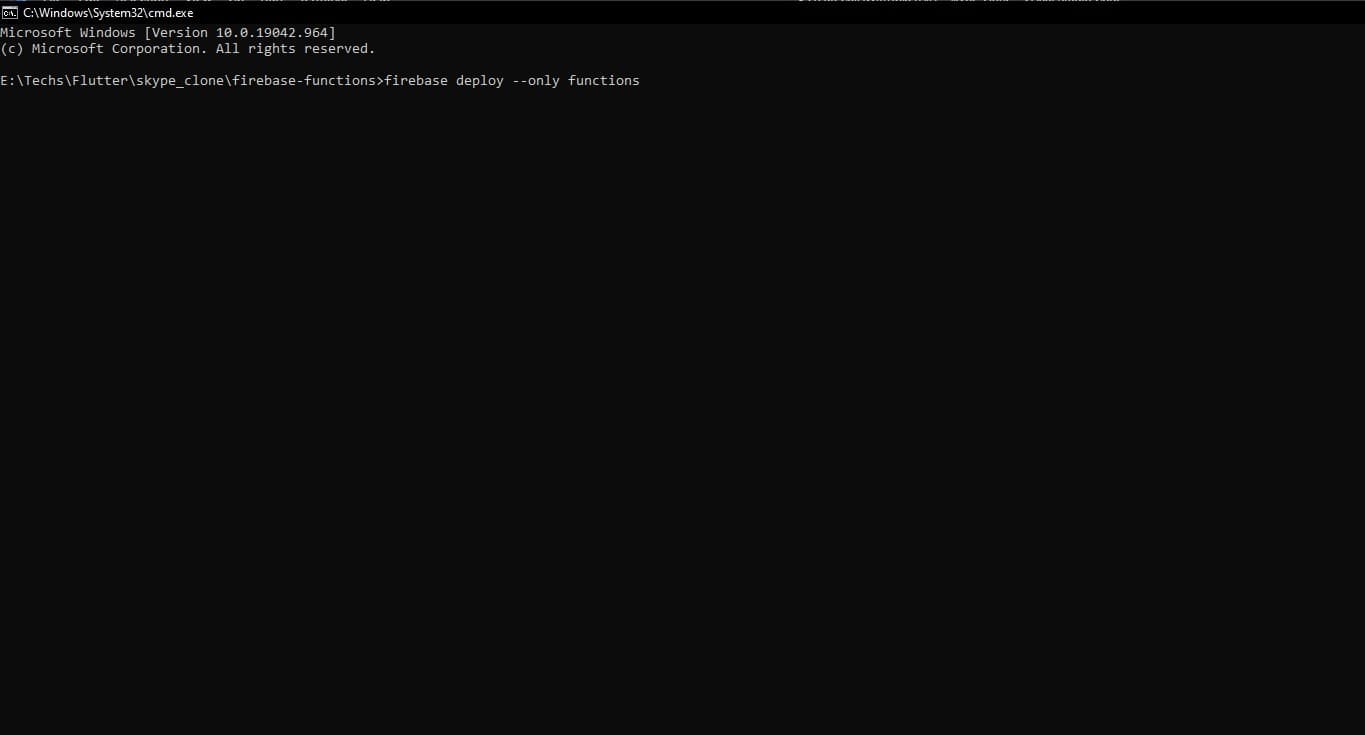
Now go to the firebase console and open cloud functions service. Then you will see newly added function.
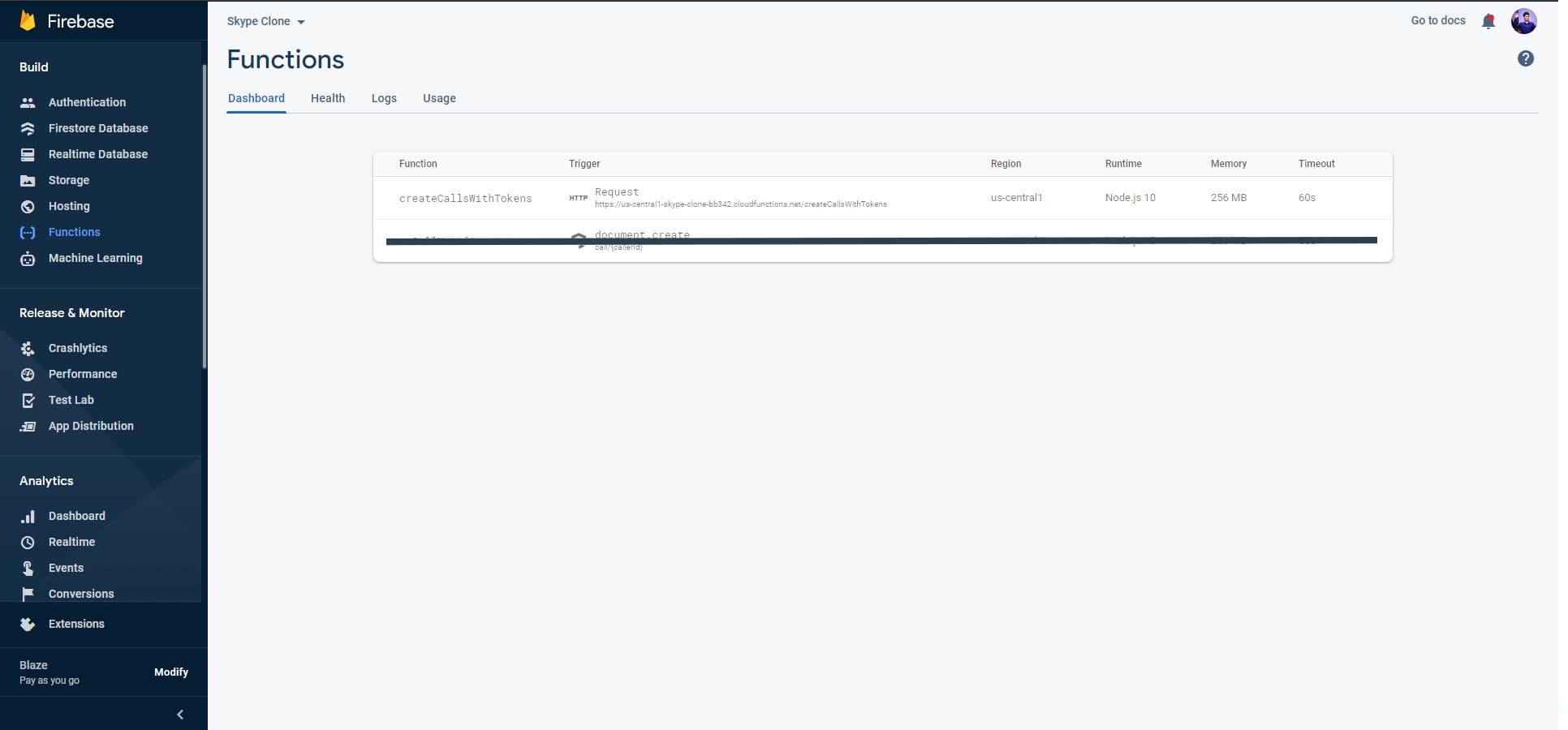
Step6- Call firebase cloud function in flutter app and create calling screen
In flutter project create a call_methods.dart file and create a class called “CallMethods”. Inside that call the firebase cloud function as below and return the token and channelId.
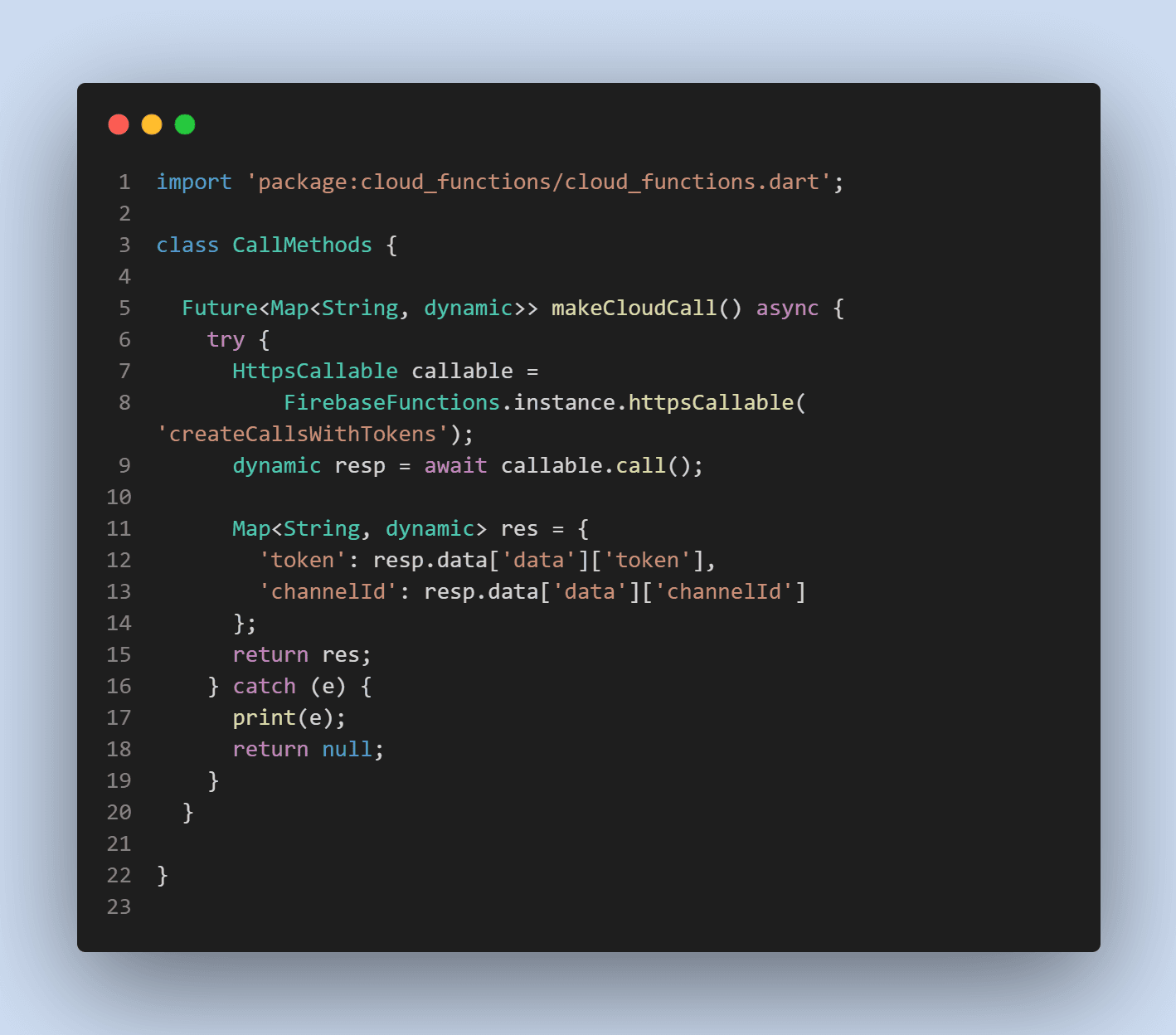
Now call this method anywhere you want.
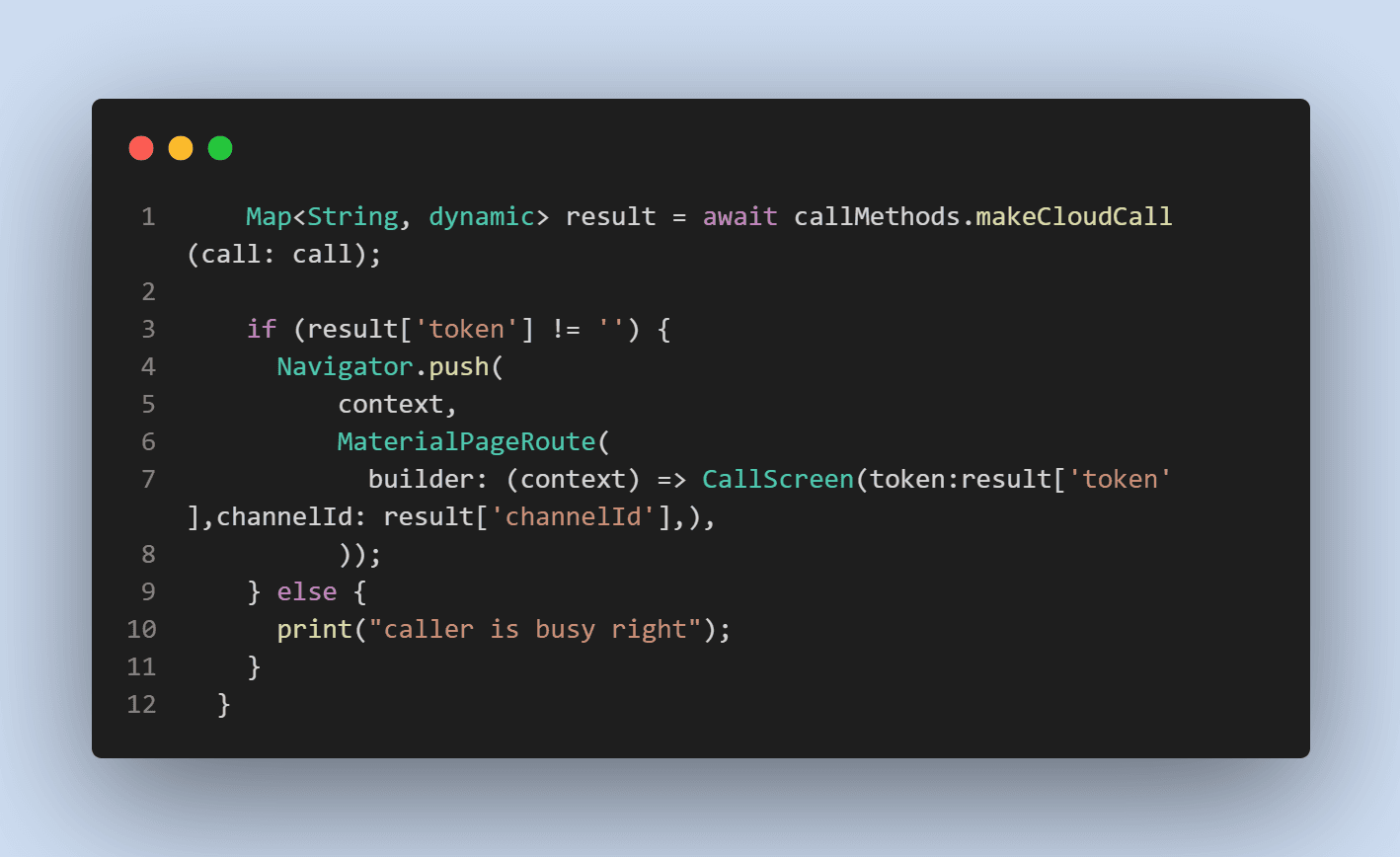
Finally add agora_rtc_engine ^3.1.3 dependency from pub.dev to pubspec.yaml file to add agora dependency to your project. Then create callScreen.dart file and implement Call Screen as below.
